반응형
오늘은 프린트 창을 따로 띄우지 않고 직접 프린터에 연결하여 이미지를 출력하는 방법을 알아보겠다.
PySide6.QtPrintSupport에서 QPrinter, QPrinterInfo를 import하고 부가적으로 Image관련 모듈들을 추가해 준다.
from PySide6.QtPrintSupport import QPrinter, QPrinterInfo
from PySide6.QtGui import QImage, QImageReader, QPainter, QPageLayout, QPageSize
반응형
코드
파일 경로와 인쇄할 인쇄 매수를 파라미터로 받아서 출력하는 함수를 작성했다.
def print_file(file_path, copy_count) :
#기본 프린터 정보 가져오기
default_printer = QPrinterInfo.defaultPrinter()
if (default_printer.isNull()) :
util.LoggerFactory._LOGGER.error('연결된 프린터가 없습니다.')
return False
else :
util.LoggerFactory._LOGGER.info('연결된 프린터 : ' + QPrinterInfo.defaultPrinterName())
#프린터 생성
printer = QPrinter(default_printer, mode=QPrinter.HighResolution)
#인쇄 매수 설정
printer.setCopyCount(copy_count)
#페이지 크기 설정 (A4)
printer.setPageSize(QPageSize.A4)
#페이지 방향 설정 (가로)
printer.setPageOrientation(QPageLayout.Orientation.Landscape)
#DPI 설정(해상도)
printer.setResolution(96)
#이미지 용량이 큰 경우 메모리 제한 해제
QImageReader.setAllocationLimit(0)
img = QImage(file_path)
scaled_img = img.scaled(printer.pageRect(QPrinter.DevicePixel).width(), printer.pageRect(QPrinter.DevicePixel).height(), aspectMode=Qt.KeepAspectRatio, mode=Qt.SmoothTransformation)
painter = QPainter()
painter.begin(printer)
painter.drawImage(0, 0, scaled_img)
painter.end()
- QPrinterInfo.defaultPrinter() 프린터 선택 UI가 없으므로 기본으로 설정된 프린터정보를 가져온다. Null 인 경우 연결된 프린터가 없다는 에러를 표시하고 있는 경우 프린터 명을 출력한다.
- QPrinter에 인자로 프린터 정보를 받아 printer 객체를 생성한다. (이 때, mode=QPrinter.HighResolution 으로 해줘야 artifact가 덜 하고 깔끔하게 나온다.)
- setCopyCount() 으로 인쇄할 매수를 설정한다.
- setPageSize() 으로 페이지 크기를 설정한다.
- setPageOrientation() 으로 인쇄 방향(가로/세로)을 설정한다. (Landscape-가로, Portrait-세로)
- setResolution() 으로 dpi(해상도)를 설정한다.
- 이미지 용량이 큰 경우 QImageReader.setAllocationLimit(0)으로 설정해주면 제한이 해제된다.
- 이미지 해상도가 용지 크기보다 큰 경우, scaled()함수를 이용하여 용지 크기에 맞게 사이즈를 조절한다. 이 때, aspectMode=KeepAspectRatio, mode=SmoothTransformation 로 설정하면 이미지 깨짐이 덜 하다.
- QPainter에 PaintDevice로 설정된 Printer를 넣어 준 후, drawImage()를 이용하여 프린트할 이미지를 그려준다. begin, end로 device를 열고 닫아주면 출력이 완료된다.
지원되는 QPageSize 목록
QPrinter::A0 | 5 | 841 x 1189 mm |
QPrinter::A1 | 6 | 594 x 841 mm |
QPrinter::A2 | 7 | 420 x 594 mm |
QPrinter::A3 | 8 | 297 x 420 mm |
QPrinter::A4 | 0 | 210 x 297 mm, 8.26 x 11.69 inches |
QPrinter::A5 | 9 | 148 x 210 mm |
QPrinter::A6 | 10 | 105 x 148 mm |
QPrinter::A7 | 11 | 74 x 105 mm |
QPrinter::A8 | 12 | 52 x 74 mm |
QPrinter::A9 | 13 | 37 x 52 mm |
QPrinter::B0 | 14 | 1000 x 1414 mm |
QPrinter::B1 | 15 | 707 x 1000 mm |
QPrinter::B2 | 17 | 500 x 707 mm |
QPrinter::B3 | 18 | 353 x 500 mm |
QPrinter::B4 | 19 | 250 x 353 mm |
QPrinter::B5 | 1 | 176 x 250 mm, 6.93 x 9.84 inches |
QPrinter::B6 | 20 | 125 x 176 mm |
QPrinter::B7 | 21 | 88 x 125 mm |
QPrinter::B8 | 22 | 62 x 88 mm |
QPrinter::B9 | 23 | 33 x 62 mm |
QPrinter::B10 | 16 | 31 x 44 mm |
QPrinter::C5E | 24 | 163 x 229 mm |
QPrinter::Comm10E | 25 | 105 x 241 mm, U.S. Common 10 Envelope |
QPrinter::DLE | 26 | 110 x 220 mm |
QPrinter::Executive | 4 | 7.5 x 10 inches, 190.5 x 254 mm |
QPrinter::Folio | 27 | 210 x 330 mm |
QPrinter::Ledger | 28 | 431.8 x 279.4 mm |
QPrinter::Legal | 3 | 8.5 x 14 inches, 215.9 x 355.6 mm |
QPrinter::Letter | 2 | 8.5 x 11 inches, 215.9 x 279.4 mm |
QPrinter::Tabloid | 29 | 279.4 x 431.8 mm |
QPrinter::Custom | 30 | Unknown, or a user defined size. |
출처 : https://doc.qt.io/archives/qt-4.8/qprinter.html#PaperSize-enum
QPrinter Class | Qt 4.8
QPrinter Class The QPrinter class is a paint device that paints on a printer. More... Note: All functions in this class are reentrant. Public Types enum ColorMode { Color, GrayScale } enum DuplexMode { DuplexNone, DuplexAuto, DuplexLongSide, DuplexShortSid
doc.qt.io
QPrinter - Qt for Python
Previous QPrintPreviewWidget
doc.qt.io
DrawImage를 쓰는 경우 이미지가 살짝 블러된것 같은 느낌으로 출력되는데 이걸 해결하느라 애먹었다. 단순하게 Printer mode를 HighResolution으로 바꿔주면되는걸 한참 걸렸다.
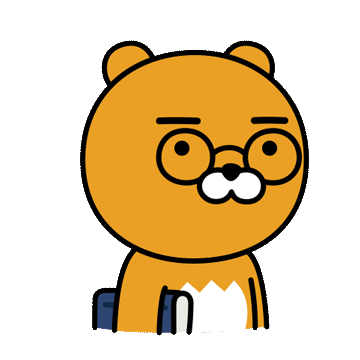
반응형
'Python > Pyside6' 카테고리의 다른 글
[Python/PySide6] QMessageBox 폰트 크기 변경 방법 (0) | 2022.10.19 |
---|---|
[Python/PySide6] qrc 리소스 파일 .py 로 변환하여 사용하는법 (0) | 2022.07.07 |
댓글