2022.08.04 - [개발 Study/Node, js, react] - [React/Redux] Redux 사용법 (1) -State/Action/Reducer/Store
[React/Redux] Redux 사용법 (1) -State/Action/Reducer/Store
Redux란? 상태 관리 라이브러리로 Component별로 props/state를 바꿔주는 복잡한 관계를 redux 하나로 외부에서 state들을 공용으로 관리하여 가져다 쓸 수 있다. 1. State(상태) State란 React Component 내에..
chuun92.tistory.com
로그인 페이지에서 지난번에 작성했던 UserReducer의 LOGIN 액션을 실행시키고 User State를 변경하여 Main 페이지에서 User State를 불러오는 예제를 보도록 하자.
1. UseDispatch
Action은 Dispatcher를 통해 실행시킬 수 있으며 Action에 변경할 새 State를 넣어줘야 Reducer에서 이전 State와 비교하여 변경사항을 적용하여 저장한다.
사용 방법은 useDispatch()로 Dispatcher를 생성하여 Action.js에서 선언했던 함수를 상태값과 함께 넣어주면 된다. 아래 코드를 참조해보자.
id로 userId를 받고 function component에 state로 선언된 nickname을 받아 user객체를 생성하여 login()함수에 넣어 action을 실행시킨다.
const dispatch = useDispatch();
const loginPostwork = (userId) => {
var user = {id : userId, nickname : nickname};
dispatch(login(user));
console.log('login success ', nickname);
navigate(`/main`, {replace : false, state : { user : nickname}});
props.HandleLocation(`/main`);
}
그럼 아래 UserReducer에서 새 user 상태 값을 받고 이전과 비교하여 새 State를 return하여 store에 저장한다.
export const LOGIN = "USER/LOGIN";
export const LOGOUT = "USER/LOGOUT";
const initialState = {
User : {
id : '',
nickname : '',
}
};
const userChecker = (state = initialState, action) => {
switch (action.type) {
case LOGIN:
return {
...state,
User : {
...state.User,
id : action.payload.id,
nickname : action.payload.nickname,
}
};
case LOGOUT:
return {
...state,
User : {
id: '',
nickname: '',
}
};
default:
return state;
}
};
export default userChecker;
2. UseSelector
UseSelector는 Redux-Store에 저장된 State 값을 반환한다.
다음으로 메인페이지에서 변경된 User State를 받아와서 읽어들이는 코드를 보자.
const userInfo = useSelector((state) => state.userReducer.User);
useSelector 함수를 이용하여 state를 받고 state는 처음에 rootReducer를 받았으므로 .userReducer로 한번 타고들어간 다음, User데이터 객체에 접근하여 user정보를 받아온다.
3. 미들웨어 Redux-logger로 확인하기
logger로 확인하면 아래와 같이 action 명 시간, 이전 State/Action/다음 State 를 Reducer 별로 객체 별로 데이터 값을 확인할 수 있다. logger는 npm install redux-logger 를 하면 설치할 수 있다. 그러나 devTools에서 보려면 redux-devtools-extension도 함께 설치해줘야한다.
logger는 아래와 같이 적용할 수 있다.
import { legacy_createStore as createStore, applyMiddleware, compose } from "redux";
import rootReducer from "./reducers/rootReducer";
import logger from "redux-logger";
import { composeWithDevTools } from "redux-devtools-extension";
// 배포 레벨에서는 리덕스 발동시 찍히는 logger를 사용하지 않습니다.
const enhancer =
process.env.NODE_ENV === "production"
? compose(applyMiddleware())
: composeWithDevTools(applyMiddleware(logger));
const store = createStore(rootReducer, enhancer);
export default store;
다음 시간엔 Redux-persist로 Session Storage에 원하는 상태 값을 저장하는걸 배워보자!!
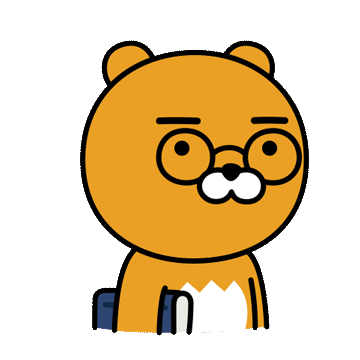
'개발 Study > React' 카테고리의 다른 글
[Error] NextJS 에러 처리 - Parsing error : Cannot find module 'Next/babel' (3) | 2022.09.01 |
---|---|
[React/Redux] Redux-persist 사용법 - (새로고침 후 상태 유지) (0) | 2022.08.08 |
[React/Redux] Redux 사용법 (1) -State/Action/Reducer/Store (0) | 2022.08.04 |
[Error] Redux createStore 에러 처리 (deprecated) (0) | 2022.08.01 |
[React] UseEffect, UseRef 사용법 (0) | 2022.07.29 |
댓글